Custom Code
Custom Code in the Visual Editor
The Builder Visual Editor helps you add event handlers, bind to state, and even add custom javascript that runs on your page
Managing state and actions
In Builder, you can bind state values to Builder blocks and implement custom actions when users interact with your content.
Buiding Interactivity Using State and Actions
state
is a javascript concept that simply says an object is in a certain state, and you can update its properties and
it's behaviour will change accordingly. State and actions make your content interactive. Below, we will show you a
simple way to use State and actions.
using State and actions
Start by dragging and dropping in a template. Add two buttons below the template. one button will make the content disappear, and the other will make he content reappear.
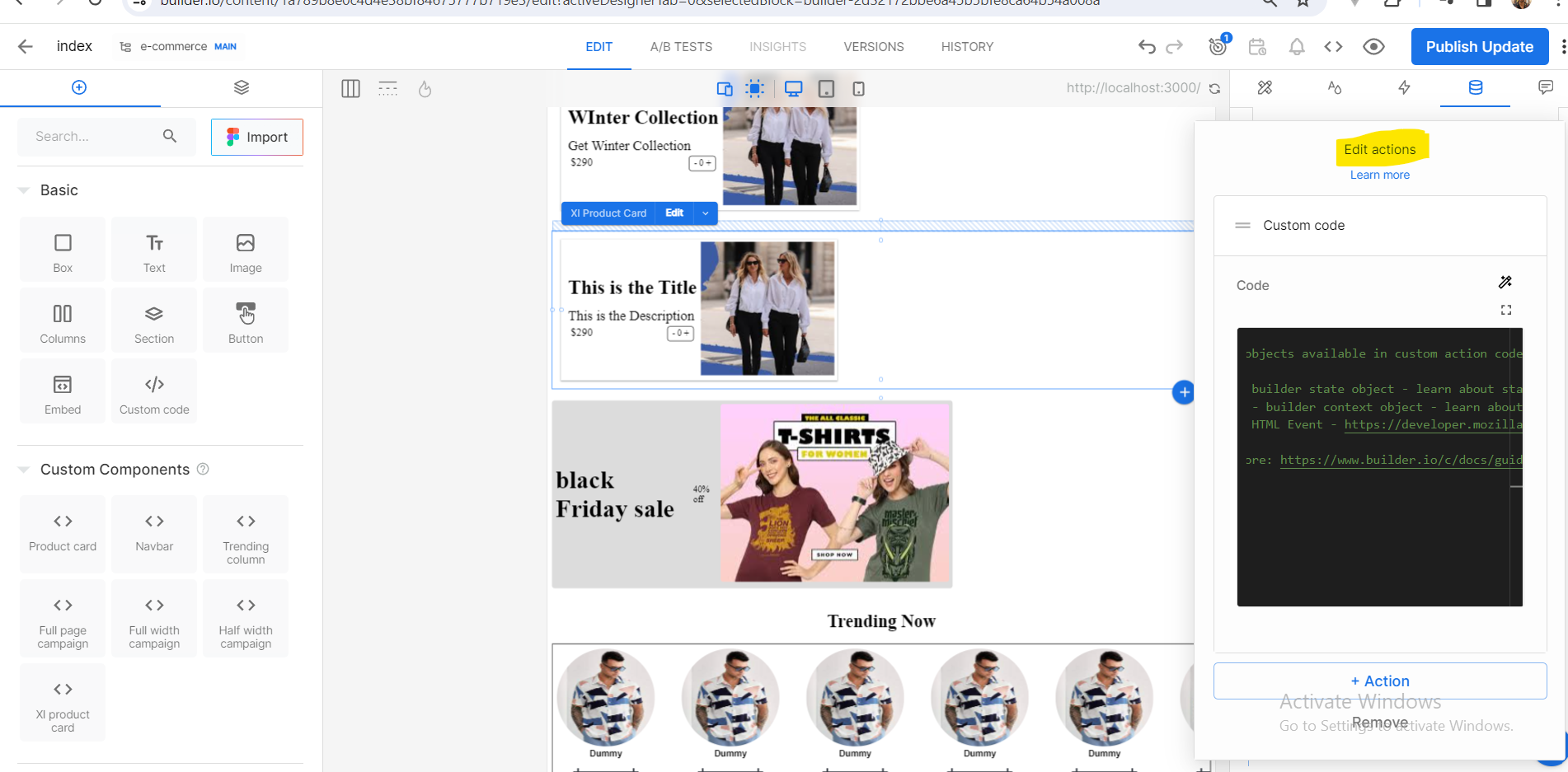
once we have our buttons in place, we are going to use the state object. click on the reappear button, naviagate to
the "Data" tab, and click "new action" in the element actions section. set the action to "click", and then click "edit
action". This is where we will use a bit of javascript. Enter in state.hidden=false
, we can add any property
name on the State object. if you aren't familiar, all you need to know is we are setting State to hidden, and then
equaling it to false ( while means no). for our other button, we are going to follow the same steps, but state will be
set to true:
state.hidden = true;
Now you can click on the buttons and see our function working. on the left side of the editor, you can see the hidden being toggled on and off when our buttons are clicked. lastly, disappear / reappear. click on the content that you want to apply the hidden property to. At the top of the 'Data' tab in the binding section, click "get" and select "hide if" Then, click "from" and select "hidden".
When adding custom javascript
code within a state binding or action handler, remember that you don't need to use
the return keyword to yield a value, instead choose from these four examples:
1. Data binding: one-line expression
For straightforward, one-line expressions, you can write code directly in the code editor without the return statement:
state.showMessage ? 'say Hello' : 'say goodbye'
2. Data Binding: complex code blocks
For more intricate logic, export a default value:
let greeting;
if (justLoggedIn) {
value = 'welcome';
} else {
value = 'hi';
}
export default greeting;
3. Accessing State
you can access Builder state in both state bindings and action handlers. While state bindings are typically closely bound to builder , you can also modify the state value within the binding.
// use the plain value from state
state.someStringValue
// uppercase the state value
state.someStringValue.toUpperCase()
// return a different value depending on state property
state.someBooleanValue ? 'yes' : 'no'
- Action handlers: using the Event object
Action handlers introduce an additional object you can access : events
.
This event
object represents the HTMLevent triggered by the selected action. you can leverage event to access
the event's target element or invoke methods such as preventDefault
or stopPropagation
:
// accessthe value attribute (ie from an input )
//and store it in builder state
state.someStateProp = event.currentTarget.value;
// if you have a link with an href, you might want to stop
// default browser navigation and handle this yourself
event.preventDefault();
var targetElementId = event.currenttarget.id;
var elemntToScroolTo = document.getElementById(targetElementId);
elementToScrollTo = document.getElementByID(targetElementID);
elementToScrollTo.scrollIntoView({behavior: 'smooth'});
Editing Custom javascript and css
To add custom styles or javascript to your content:
- In the content entry, go to the Data tab.
- Expand the code section.
- click the Edit Custom js + css button
- in the window that opens, you can put your css on the left and your javascript on the right.
Custom Javascript example
you can run js client side and/or server ide using Builder.isBrowser
and Builder.isServer
. By default,
any code not wrapped with Builder.isBrowser
or Builder.isServer
is run on Builder's Servers.
To run code client-side, wrap it in if (Builder.isBrowser){}
For example, suppose you're rendering your Builder content lazily on the client and you want to support linking directly to certain sections of a page based on the ID attribute of the section. You could do this with some custom javascript
if (Builder.isBrowser) {
const el = location.hash &&
document.getElementById(location.hash.slice(1))
el.scrollIntoView({behaviour: 'smooth'});
}
you also have access to fetch() on the client and server. For server side data, you can export a default promise that resolves with the necessary data before responding :
async function main() {
if (Builder.isServer) {
//place code that you want to only run on the server
// any data fetched on the server will be available to rehydrate on he client side if added to the state object
await fetch(someUrl)
.then(res => res.json())
.then(data => {
state.someData = data;
})
if (Builder, isBrowser) {
//code that you only want to run in the client side ( Browser)
//ex-anything that uses document, window which is not available on server side
}
}
}
export default main();
in the provided example, data fetched on the server is stored on the state
object and can be accessed as data
bindings in both the server and client UIs.
Remember to exercise caution and consider the impact on performance and security when adding custom code to your site. For instance, when incorporating custom event listeners, ensure the use of passive events for proper handling.
When adding your custom javascript code, the useful builder
values include
Builder.isServer
: Use to determine if the code is running on the server-side (such as in node.js environment) or not. it returnstrue
if the code is executing on the server, andfalse
if it's running in a browser.Builder.isBrowser
: Use to check if the code is running on the browser environment. it returntrue
if the code is executing in a web browser, andfalse
otherwise, including server-side.Builder.isPreviewing
: use to check if the code is currently in preview mode within the Visual Editor, and false if it's not in the preview mode.Builder.isEditing
: use to check of the code is currently being edited,It returnstrue
and false if it's not being edited.